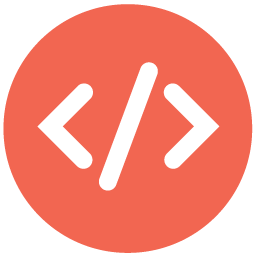
Simple vanilla javascript filter
18 Mar 2016
In this post I’ll show you how to implement a simple filter in vanilla javascript, like this one:
- Blue Bird
- Blue Cat
- Red Rabbit
- Green Chicken
- Blue Dog
- Red Cow
- Red Frog
- Green Bee
- Blue Dolphin
- Green Octopus
- Red Iguana
- Green Human
Let’s say that we have a very long list of colorful animals like the following:
<ul id="colorful-animals-list">
<li data-color="blue" >Blue Bird</li>
<li data-color="blue" >Blue Cat</li>
<li data-color="red" >Red Rabbit</li>
<li data-color="green">Green Chicken</li>
<li data-color="red" >Red Dog</li>
<li data-color="red" >Red Cow</li>
<li data-color="green">Green Pig</li>
</ul>
And we want to be able to filter animals by color (because otherwise it would be really difficult to find the ones we are looking for).
The algorithm:
For hiding the elements, we will change their display
property to none
.
So we will implement the function Refresh
to perform this task.
We have to create a select input with the values we want to filter. Something like this:
<select id="color-select" name="color-select">
<option value=""></option>
<option value="red">Red</option>
<option value="green">Green</option>
<option value="blue">Blue</option>
</select>
And add an event listener for that select input, so that it triggers the Refresh
function every time it changes.
Here is the complete code:
var elements = document.
getElementById('colorful-animals-list').
children,
length = elements.length,
select = document.getElementById('color-select');
Refresh = function () {
var selected_color = select.value;
for (var i = 0; i < length; i++) {
elements[i].style.display =
(!selected_color ||
elements[i].dataset.color === selected_color
) ? '' : 'none';
}
};
select.addEventListener('change', function(event) {
Refresh();
event.preventDefault();
});
That’s it! Check out the repo.